Backend API quick start
This article describes how to implement Fraud Prevention in your application using a backend API approach. Initially, you need to install and initialize the relevant Mosaic's SDK (for Web, Android, or iOS) in order to collect and stream telemetry data to Mosaic. The backend API then processes this data and responds accordingly, reporting specific user actions to Mosaic and fetching risk assessment recommendations for those actions.
Note
Backend integration is a recommended integration for production environments. Learn more about integration options: Client-side integration vs Backend integration.
How it works
The flow starts with the user navigating to the webpage or app (1). The SDK gets initialized, obtains a session token, and starts sending telemetry to Mosaic (2). When a user performs an action, for example, clicks a login button (3), your client passes this information to your backend (4) and the backend triggers an action event to Mosaic (5). Having received an action token (6), the application backend uses it to fetch recommendation from Mosaic (7 & 8) and instructs the client to act accordingly (9) in order to complete the login procedure (10). As a last step, your server can report the action result back to Mosaic and set the user (11).
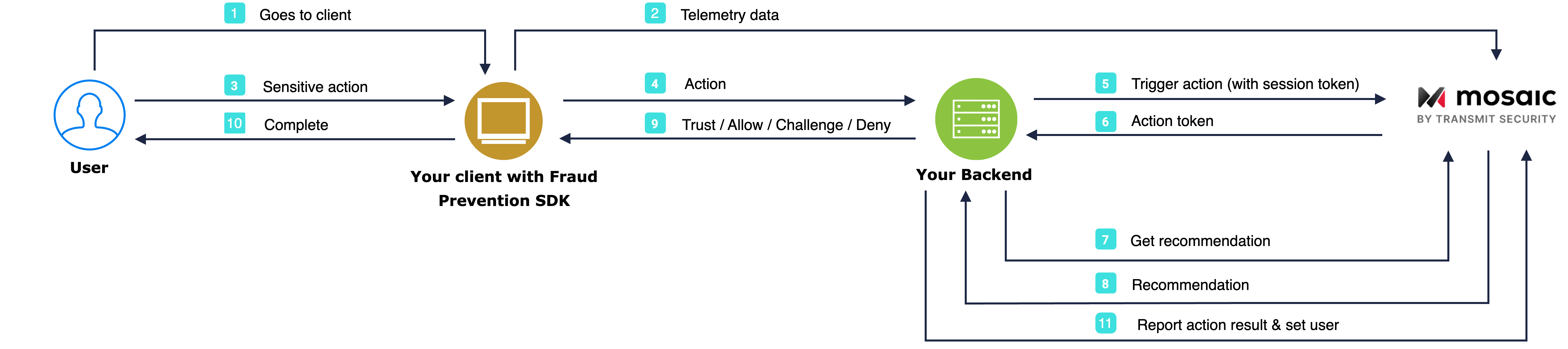
Step 1: Get client credentials
Client credentials are used to identify your app and generate access tokens for authorizing Mosaic requests. To obtain them, you'll need to create an application in the Admin Portal (if you don’t have one yet).
- From Applications , click Add application .
- Add the friendly application name to display in the Admin Portal.
-
Add an OIDC client, specify the client secret as an authentication method, and add your website URL as a redirect URI (e.g.,
https://your-domain.com
). Adding a client automatically generates client credentials.Note
These fields are required for all Mosaic apps, but won’t be used for Fraud Prevention.
Step 2: Start an SDK session
To detect risk and generate recommendations, Fraud Prevention relies on the analysis of telemetry data, which includes details about user interactions, journeys, and device information. To transfer this data to Mosaic, you need to use Mosaic's client-side SDK. Upon initialization, the SDK automatically tracks telemetry data and streams it to Mosaic.
For instructions on how to load and initialize SDKs, refer to:
- Web : Step 2 in the Javascript quick start guide
- Android : Step 2 & 3 in the Android quick start guide
- iOS : Step 2 & 3 in the iOS quick start guide
Upon initialization, the SDK generates a session token, which should be passed to your backend as it will later be used to link your backend calls to the session.
<script>
document.getElementById("ts-platform-script").addEventListener("load", async function() {
await window.tsPlatform.initialize({
clientId: "[CLIENT_ID]",
drs: {
enableSessionToken: true,
serverPath: "https://api.transmitsecurity.io/risk-collect/", // For EU, use "https://api.eu.transmitsecurity.io/risk-collect/"; for Canada, use "https://api.ca.transmitsecurity.io/risk-collect/"
}
});
// Retrieves session token required for backend API calls
const sessionToken = await window.tsPlatform.drs.getSessionToken();
});
</script>
TSAccountProtection.getSessionToken(object : ISessionTokenCallback {
override fun onSessionToken(sessionToken: String) {
}
})
TSAccountProtection.getSessionToken { token in
debugPrint("[DEBUG]: Fraud Prevention session token: \(token)")
}
Step 3: Get access token
Mosaic APIs are authorized using an OAuth access token so you'll need to fetch a token using your client credentials (from step 1). The token should target the following resource: https://risk.identity.security
. To do this, send the following request:
const { access_token } = await fetch(
`https://api.transmitsecurity.io/oidc/token`,
{
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
}
body: new URLSearchParams({
grant_type: client_credentials,
client_id: [CLIENT_ID],
client_secret: [CLIENT_SECRET],
resource: 'https://risk.identity.security'
})
}
);
Step 4: Trigger actions
To obtain risk recommendations for sensitive actions, your application should report these actions using a backend API. To do this, send a POST request to /action/trigger-action like the one below when a user requests to perform the action. Replace [ACTION_TYPE]
with the appropriate action type from our list of actions. To improve Fraud Prevention, pass the correlation ID and user ID/claimed user ID (optional). This API returns an action_token
to be used in the next step to fetch a recommendation.
User identity
Depending on the user authentication status—whether or not the user has already authenticated—the POST request to /action/trigger-action can optionally include one of the following identifiers:
-
user_id
(for authenticated users): Reporting auser_id
sets the user for this action . To set the user for session and all subsequent events, see Report action result . -
claimed_user_id
(for users that haven't authenticated yet)
Note that the user identifier should be an opaque identifier for the user in your system. This must not include personal user identifiers, such as email, in plain text.
Here is an example:
import fetch from 'node-fetch';
async function run() {
const query = new URLSearchParams({
get_recommendation: 'false' // Default. Set to true to return the recommendation and skip Step 5
}).toString();
const resp = await fetch(
`https://api.transmitsecurity.io/risk/v1/action/trigger-action`,
{
method: 'POST',
headers: {
'Content-Type': 'application/json',
Authorization: 'Bearer [ACCESS_TOKEN]' // Access token generated in Step 3
},
body: JSON.stringify({
session_token: '[SESSION_TOKEN]', // Session token generated by SDK in Step 2
action_type: '[ACTION_TYPE]', // For example, login
correlation_id: '[CORRELATION_ID]', // Optional
user_id: '[USER_ID]', // Optional. Use only if user has authenticated.
claimed_user_id: '[CLAIMED_USER_ID]' // Optional
})
}
);
const data = await resp.json();
console.log(data);
}
run();
Optimization
Setting get_recommendation
to true
allows you to obtain a risk recommendation without calling Get recommendation API (Step 5).
Step 5: Fetch recommendation
You can fetch recommendations for the reported action from your backend. To do this, invoke the Recommendation API by sending a request like the one below. Replace [ACTION_TOKEN]
with the token returned in Step 4.
const query = new URLSearchParams({
action_token: '[ACTION_TOKEN]', // Action token returned in step 4
}).toString();
const resp = await fetch(
`https://api.transmitsecurity.io/risk/v1/recommendation?${query}`,
{
method: 'GET',
headers: {
Authorization: 'Bearer [ACCESS_TOKEN]', // Access token generated in step 3
},
}
);
Step 6: Report action result
Report the action outcome back to Mosaic to enrich the risk profile. By reporting the action result, you help improve risk detection algorithms, that especially makes sense for "challenge" recommendations. For example, in Step 5 Mosaic returned "challenge" recommendation, and your application asked a user to authenticate with SMS OTP which they successfully did. This could a sign of a legitimate action.
User identity
Along with the action result, a user identifier can be reported to Mosaic. Reporting a user_id
on a successful authentication event, sets the user for all subsequent events coming from this device in the current device session, or until this device needs the user to re-login again as part of the application flow, or until the user is explicitly cleared.
Reporting user_id
for other action types or unsuccessful authentication attempts doesn't persist the user.
Note that the user identifier should be an opaque identifier for the user in your system. This must not include personal user identifiers, such as email, in plain text.
import fetch from 'node-fetch';
async function run() {
const resp = await fetch(
`https://api.transmitsecurity.io/risk/v1/action/result`,
{
method: 'POST',
headers: {
'Content-Type': 'application/json',
Authorization: 'Bearer [ACCESS_TOKEN]', // Access token generated in step 3
},
body: JSON.stringify({
action_token: '[ACTION_TOKEN]', // Action token returned in step 4
result: '[RESULT]', // Result of action. One of success, failure, or incomplete
user_id: '[USER_ID]', // Optional. If provided, sets the user for device session
challenge_type: '[CHALLENGE]' // Optional. Specify the type of challenge performed such as passkey or sms_otp
})
}
);
const data = await resp.json();
console.log(data);
}
run();
Step 7: Clear user
The user gets automatically cleared in the following cases:
- Once a device session expires
- Triggering a new login action
- Reporting a different user ID for an event
-
Calling
clearUser()
from the client side
Note
Triggering a logout
action does not automatically clear the user.